Android Studio TextView Tutorial with Examples
A TextView is a GUI component that consists of text. TextViews are used to display text to the user.
Example of TextView in XML
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
tools:context=".MainActivity">
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="This is display text to the user"/>
</LinearLayout>
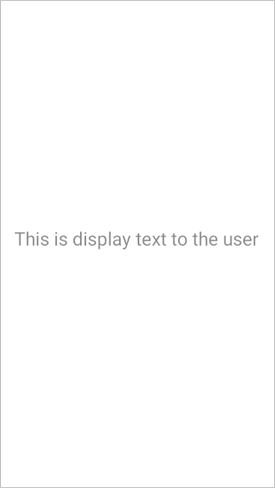
Attributes of TextView
android:layout_width - This attribute is used to set width of a TextView. It is required attribute.
Values of layout_width attribute :
match_parent : It will set the TextView width according to the parent layout's width. For example, if the width of a parent layout is 200dp then the width of a TextView is also 200dp.
wrap_content : It will set the TextView width according to the length of text in the TextView. TextView width is also increased or decreased when its text is increased or decreased
other : You can also set width in dp or px like 200dp, 50px, etc. It will set width according to your device's screen resolution.
android:layout_height - This attribute is used to set height of a TextView. It is required attribute.
Values of layout_height attribute :
match_parent : It will set the TextView height according to the parent layout's height. For example, if the height of a parent layout is 200dp then the height of a TextView is also 200dp.
wrap_content : It will set the TextView height according to the length of text in the TextView. TextView height is also increased or decreased when its text is increased or decreased
other : You can also set height in dp or px like 200dp, 50px, etc. It will set height according to your device's screen resolution.
TextView Margin Attributes
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_marginStart="50dp"
android:layout_marginTop="20dp"
android:layout_marginEnd="50dp"
android:layout_marginBottom="20dp"
android:background="#1A000000"
android:text="This is display text to the user" />

android:layout_marginStart - This attribute is used to give extra space from left side of the TextView.
Values of layout_marginStart attribute :
You can set its value in dp or px like 20dp, 50px, etc.
android:layout_marginEnd - This attribute is used to give extra space from right side of the TextView.
Values of layout_marginEnd attribute :
You can set its value in dp or px like 20dp, 50px, etc.
android:layout_marginTop - This attribute is used to give extra space from top of the TextView.
Values of layout_marginTop attribute :
You can set its value in dp or px like 20dp, 50px, etc.
android:layout_marginBottom - This attribute is used to give extra space from bottom of the TextView.
Values of layout_marginBottom attribute :
You can set its value in dp or px like 20dp, 50px, etc.
android:layout_margin - This attribute is used to give extra space from every side (left, right, top, bottom) of the TextView.
Values of layout_margin attribute :
You can set its value in dp or px like 20dp, 50px, etc.
TextView Padding Attributes
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:paddingStart="50dp"
android:paddingEnd="20dp"
android:paddingTop="50dp"
android:paddingBottom="20dp"
android:text="This is display text to the user" />

android:paddingStart - This attribute is used to give inside space between text and left side of TextView edge.
Values of paddingStart attribute :
You can set its value in dp or px like 20dp, 50px, etc.
android:paddingEnd - This attribute is used to give inside space between text and right side of TextView edge.
Values of paddingEnd attribute :
You can set its value in dp or px like 20dp, 50px, etc.
android:paddingTop - This attribute is used to give inside space between text and top side of TextView edge.
Values of paddingTop attribute :
You can set its value in dp or px like 20dp, 50px, etc.
android:paddingBottom - This attribute is used to give inside space between text and every side of TextView.
Values of paddingBottom attribute :
You can set its value in dp or px like 20dp, 50px, etc.
android:padding - This attribute is used to give inside space between text and every side of TextView edge.
Values of padding attribute :
You can set its value in dp or px like 20dp, 50px, etc.
TextView Text Style Attributes
android:text - This attribute is used to write text on a TextView.
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="This is text" />
Values of text attribute :
You can set any String value like "Learnoset", "Coding", "Android", etc.
android:textColor - This attribute is used to change text color of a TextView.
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="15sp"
android:textColor="#D50000"
android:text="This is a TextView with red text" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="15sp"
android:textColor="#2962FF"
android:text="This is a TextView with blue text" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="15sp"
android:textColor="#FF6D00"
android:text="This is a TextView with orange text" />
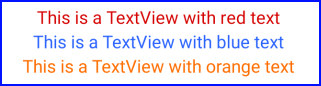
Values of textColor attribute :
You can use color codes like "#000000", "#FFFFFF", etc. or you can also get value from colors.xml file like "@color/black"
android:textSize - This attribute is used to change text size of a TextView.

Values of textSize attribute :
You can set text size in dp, px, sp like 10dp, 20px, 18sp, etc.
android:textStyle - This attribute is used to set style of text on a TextView like bold, italic, normal.
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="15sp"
android:text="This is a normal style TextView" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="15sp"
android:textStyle="bold"
android:text="This is a bold style TextView" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="15sp"
android:textStyle="italic"
android:text="This is a italic style TextView" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="15sp"
android:textStyle="bold|italic"
android:text="This is a bold|italic style TextView" />

Values of textStyle attribute :
bold : To make text bold
italic : To make text italic
normal : To make text normal (remove italic and bold style)
combined : You can also use multiple values like bold|italic by using '|' symbol in between.
android:textAllCaps - This attribute is used to make text in UPPERCASE or lowercase.
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="15sp"
android:textAllCaps="true"
android:text="This is uppercase textview" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:textSize="15sp"
android:textAllCaps="false"
android:text="This is lowercase textview" />

Values of textAllCaps attribute :
true : Text in uppercase
false : Text in lowercase
TextView Other Attributes
android:background - This attribute is used to set background of a TextView
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="#000000"
android:textColor="#FFFFFF"
android:text="TextView with black background and white text color" />
<TextView
android:layout_marginTop="10dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="#D50000"
android:textColor="#FFFFFF"
android:text="TextView with red background and white text color" />
<TextView
android:layout_marginTop="10dp"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:background="#2962FF"
android:textColor="#FFD600"
android:text="TextView with blue background and yellow text color" />
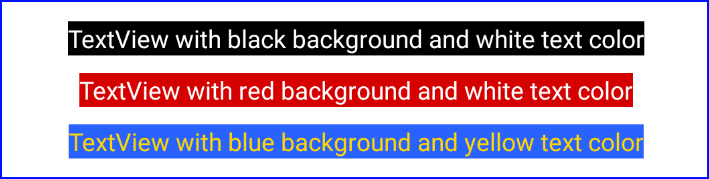
Values of background attribute :
You can use a color as TextView background like "#000000", "#FFFFFF", @color/black
You can also use an image from drawable folder as TextView background like @drawable/you_image_name
android:drawableStart - This attribute is used set an image on left side of a TextView
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:drawableStart="@mipmap/ic_launcher"
android:text="TextView with left side image" />

Values of drawableStart attribute :
You can also use an image from drawable folder like @drawable/you_image_name
Note: Use small images with resolution of 24px or 36px.
android:drawableEnd - This attribute is used to set an image on right side of a TextView
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:drawableEnd="@mipmap/ic_launcher"
android:text="TextView with right side image" />
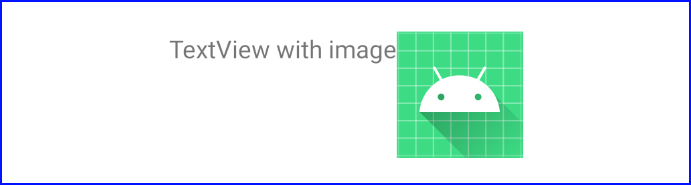
Values of drawableEnd attribute :
You can also use an image from drawable folder like @drawable/you_image_name
Note: Use small images with resolution of 24px or 36px.
android:drawableTop - This attribute is used to set an image on top side of a TextView
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:drawableTop="@mipmap/ic_launcher"
android:text="TextView with top side image" />
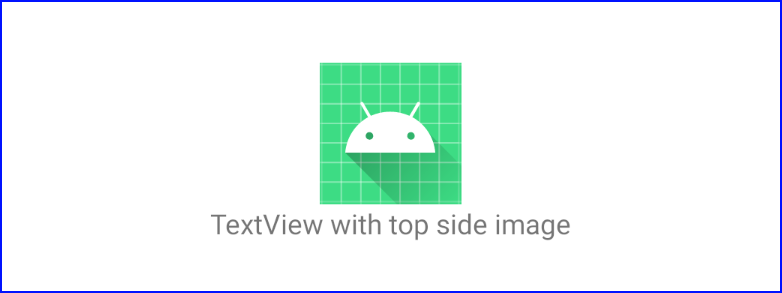
Values of drawableTop attribute :
You can also use an image from drawable folder like @drawable/you_image_name
Note: Use small images with resolution of 24px or 36px.
android:drawableBottom - This attribute is used set an image on bottom side of a TextView
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:drawableBottom="@mipmap/ic_launcher"
android:text="TextView with bottom side image" />
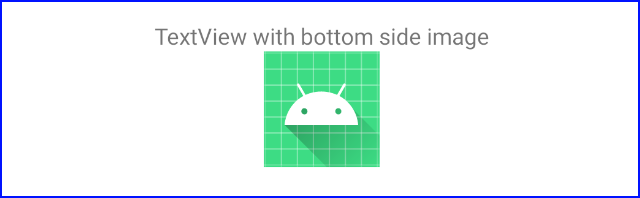
Values of drawableBottom attribute :
You can also use an image from drawable folder like @drawable/you_image_name
Note: Use small images with resolution of 24px or 36px.
Code Examples
activity_main.xml
<?xml version="1.0" encoding="utf-8"?>
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:gravity="center"
android:orientation="vertical"
tools:context=".MainActivity">
<TextView
android:id="@+id/normalTextView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="This is Normal Text"
android:textSize="18sp" />
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:background="@android:color/holo_red_light"
android:text="Text with Red BackGround and white Text"
android:textColor="#FFFFFF"
android:textSize="18sp" />
<TextView
android:layout_width="wrap_content"
android:layout_height="55dp"
android:layout_marginTop="10dp"
android:background="@drawable/round_corner_2"
android:gravity="center"
android:text="Text with Round Corner and center gravity"
android:textSize="18sp" />
<TextView
android:layout_width="wrap_content"
android:layout_height="55dp"
android:layout_marginTop="10dp"
android:gravity="center"
android:letterSpacing="0.2"
android:text="TextView with letterSpacing"
android:textSize="18sp" />
<TextView
android:layout_width="200dp"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:gravity="center"
android:text="This is Line 1 \n This is line 2 \n this is line 3"
android:textSize="18sp" />
<TextView
android:layout_width="200dp"
android:layout_height="wrap_content"
android:layout_marginTop="10dp"
android:ellipsize="end"
android:gravity="center"
android:maxLines="1"
android:text="Ths is single line TextView"
android:textSize="18sp" />
</LinearLayout>
drawable/round_corner_2
<?xml version="1.0" encoding="utf-8"?>
<shape xmlns:android="http://schemas.android.com/apk/res/android"
android:shape="rectangle">
<!--Button Color-->
<solid android:color="@color/teal_200" />
<!---Make Round Corner with radius. you can increase or decrease radius according to your need-->
<corners android:radius="100dp"/>
</shape>
MainActivity.java
import android.graphics.Color;
import android.graphics.Typeface;
import android.os.Bundle;
import android.view.View;
import android.widget.TextView;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// getting TextViews from xml file
final TextView normalTextView = findViewById(R.id.normalTextView);
// setting text programmatically
normalTextView.setText("This is Text");
// setting background color programmatically
normalTextView.setBackgroundColor(Color.RED);
// OR setting color from res/values/color file
normalTextView.setBackgroundColor(getResources().getColor(R.color.your_color));
// OR setting color direct from color code
normalTextView.setBackgroundColor(Color.parseColor("#FFFFFF"));
// setting text size programmatically
normalTextView.setTextSize(20);
// setting text style (BOLD) programmatically
Typeface typeface = Typeface.create(normalTextView.getTypeface(), Typeface.BOLD);
normalTextView.setTypeface(typeface);
// setting text style (ITALIC) programmatically
Typeface typeface2 = Typeface.create(normalTextView.getTypeface(), Typeface.ITALIC);
normalTextView.setTypeface(typeface2);
// TextView click listener
normalTextView.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View view) {
// handle TextView click...
}
});
}
}
Output

If you have any Questions or Queries
You can mail us at info.learnoset@gmail.com
Follow us to learn Coding and get in touch with new Technologies.